最近在学Java咖啡的制作方法,但是我感觉我在成为手冲大师的路上越走越远来着(难绷
此博客持续更新,旨在记录一些重点、易错点、遗忘点以及二次元美图。
命名の法
类 Class - 帕斯卡法 PascalNamingConversion
1 2 3 4
| CargoNum SpeedOfCar MyBaby HighGrade
|
方法 Method - 驼峰法 camelNamingConversion
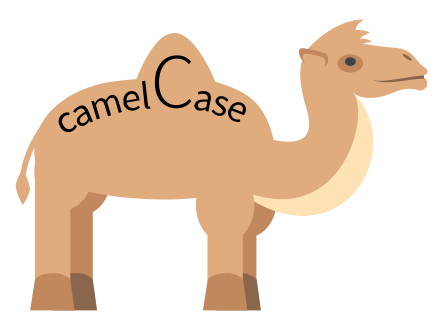
1 2 3
| positiveGravity localPeopleAge bookPage
|
数据类型
基本类型 Primitive Types
该数据类型含 byte short int float boolean char \t
等等 该类型数据按值传递,例如下面代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| package com.eg;
public class Main {
public static void main(String[] args) {
int a = 1; int b = 2; a = 5;
System.out.println(b);
} }
|
该代码先使a = 1
,而后令b = a
,即 b值与当前a值同为1。 随后变更a为5,但注意基本数据类型按值传递,所以 b仍为a的初始值1。
上面代码运行结果:
1 2 3
| 1
Process finished with exit code 0
|
封装(引用)类型 Reference Types
该类型数据按引用(地址)传递,例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| package com.eg; import java.awt.*;
public class LearnOne {
public static void main(String[] args) {
Point point1 = new Point(1, 1); Point point2 = point1; point1.x = 4; point1.y = 6;
System.out.println(point2);
} }
|
1 2 3 4
| java.awt.Point[x=4,y=6]
Process finished with exit code 0
|
生命流控制流
if-else
语句
以下代码展示如何对两个用户输入的数字作比较,并输出结果:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| package com.learn; import java.util.Scanner;
public class LearnTwo { public static void main(String[] args) { Scanner scan = new Scanner(System.in);
System.out.println("First number: "); int first = Integer.parseInt(scan.nextLine());
System.out.println("Another number: "); int second = Integer.parseInt(scan.nextLine());
if (first == second) { System.out.println("The same!"); } else if (first > second) { System.out.println("Former's larger than latter!"); } else { System.out.println("Latter's larger than former!"); }
} }
|
代码逻辑几乎等同于C
语言中的同等语句,但是代码语句的缩进大有不同,请看:
C
1 2 3 4 5 6 7 8 9
| if (conditionOne) { do statementOne; } else if (conditionTwo) { do statementTwo; } else { do statementDefault; }
|
Java
1 2 3 4 5 6 7
| if (conditionOne) { do statementOne; } else if (conditionTwo) { do statementTwo; } else { do statementDefault; }
|
while
语句
While
语句超级实用!!!
1 2 3 4
| while (_expression_) { do statements; ... }
|
(在while
中嵌套if-else
时,continue
往往可以忽略)
列表
声明和添加项
用ArrayList<*DataType*> *listName* = new ArrayList<>()
创建列表,并用*listName*.add()
方法向列表中添加项目:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| import java.util.ArrayList;
public class ArrayDemo {
public static void main(String[] args) {
ArrayList<Integer> list = new ArrayList<>(); list.add(1);
ArrayList<Double> list = new ArrayList<>(); list.add(4.2);
ArrayList<Boolean> list = new ArrayList<>(); list.add(true);
ArrayList<String> list = new ArrayList<>(); list.add("String is a reference-type variable");
} }
|
数据被存入list
后,会被隐式转换为ArrayList<>
中声明的类型。
删除项
用*listName*.remove()
方法删除特定项。
列表长度
用*listName*.size()
方法确定列表长度:
1 2 3 4 5 6 7 8 9 10
| ArrayList<String> wordList = new ArrayList<>();
wordList.add("First"); wordList.add("Second");
System.out.println(wordList.size());
2
|
判斷項的存在
用*listName*.contains()
方法確定列表中是否存在某一特定項目,該方法輸出的是布爾數(true
,false
):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| ArrayList<String> list = new ArrayList<>();
list.add("First"); list.add("Second"); list.add("Third");
System.out.println("Is the first found? " + list.contains("First"));
if (list.contains("Second")) { System.out.println("Second can still be found"); }
Is the first found? true Second can still be found
|
数组
声明
1
| int[] numbers = new int[3];
|
字符串
比较两个字符串
使用equals
方法比较两个字符串(不能用==!!):
1 2 3 4 5 6 7 8 9 10
| String one = "Ello gays"; String two = "Hello owl";
if (one.equals(two)) { System.out.println("Two string's are same!") }
Two string's are same!
|
分割字符串
用*name*.split(" *分割界限* ")
方法:
1 2 3 4 5 6 7 8 9 10 11 12 13
| String text = "first_second_third_fourth"; String[] pieces = text.split("_");
for (String block : pieces) { System.out.println(block); }
first second third fourth
|
注意分割后的字符要存放在一个数组里!!
判断字符串内包含情况
用*name*.contain(" *包含内容* ")
方法判断一个字符串内是否有想要的内容,下面的示例判断了一个字符串内是否含有”av”:
1 2 3 4 5 6 7
| String text = "lava";
if (text.contains("av")) { System.out.println("av was found"); } else { System.out.println("av not found"); }
|
定位某个字符
1 2 3 4 5 6 7
| String text = "Hello world!"; char character = text.charAt(0); System.out.println(character);
H
|
面向对象编程
备忘
Fun Fact
在IDEA中调用valueOf
方法时,IDE给出了下面的建议:

从网上搜寻得知,valueOf
内部就用了parseInt
,区别在于 parseInt
直接返回原始int
类型数据;而valueOf
又做了封装,返回Integer
类型。
还有一个区别,parseInt
期待的输入是String
,而valueOf
不是。
综上所述,一般用parse
方法取代valueOf
,除非要返回Integer
类型,不然还有封装拆箱,性能多少会耗费些。
还有,在编写计算赠与税的程序时,IDE对下面的程序块提出了优化建议:
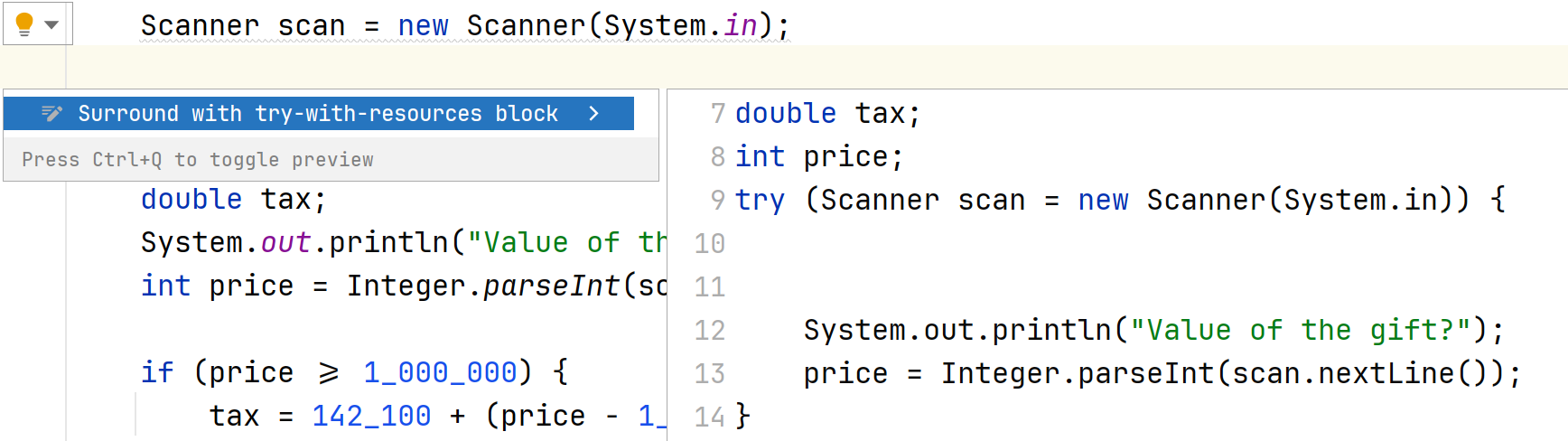
搜寻之,遂得此:
try-with-resources
语句是一个 try
语句,它声明一个或多个资源。资源 是在程序完成后必须关闭的对象。try-with-resources
语句确保在语句结束时关闭每个资源。
无论 try
语句是否正常完成或中断,它都将被关闭。
综上,对于内存敏感型的程序来说,在源码内使用try-with-resources
语句会更节省寸土寸金的内存(Are u winning, Apple?)。
输出东西
平方
1
| double twoSquareTwo = Math.pow(2, 2)
|
读取输入东西并转为字符串
1 2
| Scanner scanner = new Scanner(System.in) String input = scanner.nextLine()
|
读取输入的整数/小数/布尔数
一般使用valueOf
方法,但是作为高级程序员的初级形态(bushi),我们选择更高级的parse
方法:
1 2 3 4
| String stringInput = "</>"; int intVal = Integer.parseInt(stringInput); double dbVal = Double.parseDouble(stringInput); boolean booVal = Boolean.parseBoolean(stringInput);
|
(蜜汁)反转判断
用!
反转其后的判断条件,比如:
1 2 3 4 5 6 7 8 9 10 11
| int number = 7;
if (!(number > 4)) { System.out.println("Number is no greater than 4."); } else { System.out.println("Number is greater than 4.") }
Number is greater than 4.
|
这里的!(number > 4)
等同于(number <= 4)
。
定位列表/数组元素
切记数组从0存储数据,而size
从1开始,所以
index = numbers.size() - 1
判断空数组/列表
1 2 3 4 5 6 7
| if (input.isEmpty()) { break; }
if (input.equals("")) { break; }
|
异题
“编写一个方法public static String word()
。该方法返回一个字符串。”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| public class StringOutPut {
public static void main(String[] args) { String output = "op"; word(output); } public static void word(String str) { System.out.println(str); } }
op
|
“使程序对用户输入的数字进行搜索,如果数组含该数字,输出该数字以及其位置;否则告知number wasn't found
。”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| public static void main(String[] args) { Scanner scanner = new Scanner(System.in); int[] array = new int[10]; array[0] = 6; array[1] = 2; array[2] = 8; array[3] = 1; array[4] = 3; array[5] = 0; array[6] = 9; array[7] = 7;
System.out.print("Search for? "); int num = Integer.valueOf(scanner.nextLine()); boolean isFound = false;
for (int i = 0; i < array.length; i++) { if (num == array[i]) { System.out.println(num + " is at index " + i); isFound = true; } }
if (!isFound) { System.out.println(num + " was not found."); } }
|
输出所给csv文件中的最长名和平均年龄
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| Scanner scanner = new Scanner(System.in); int yearOfBirth = 0; int count = 0; int nameLenght = 0; String name = null; while (true) {
String input = scanner.nextLine(); if (input.equals("")) { break; }
String[] parts = input.split(",");
if (parts[0].length() > nameLenght) { nameLenght = parts[0].length(); name = parts[0]; } yearOfBirth = yearOfBirth + Integer.valueOf(parts[1]); count = count + 1; } System.out.println("Longest name: " + name); if (count > 0) { System.out.println("Age average: " + (1.0 * yearOfBirth / count)); } else { System.out.println("No input."); }
|
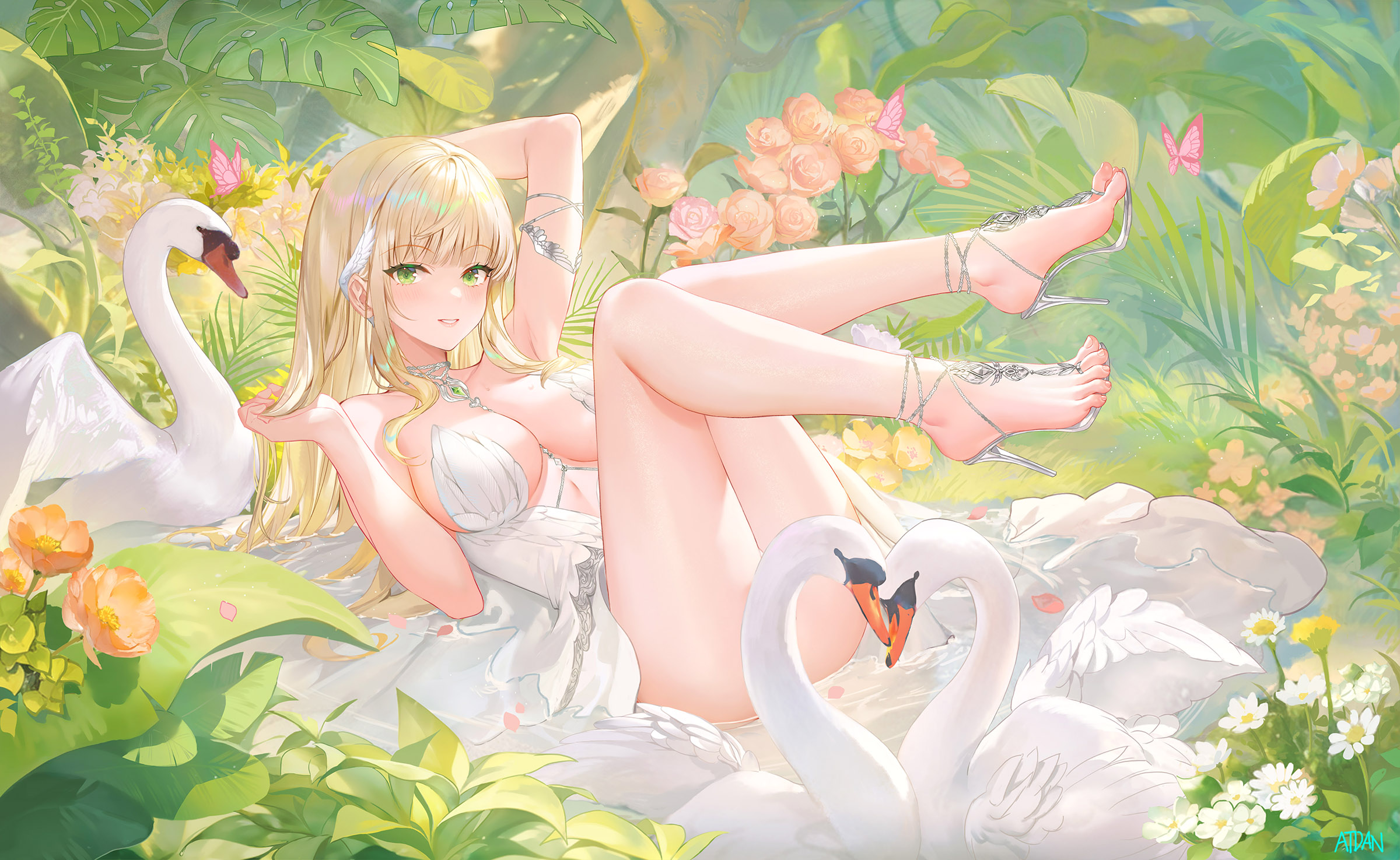